Community Widgets
Seamlessly integrate real-time data such as top community holdings, trending posts, or top-rated members directly into your site's architecture.
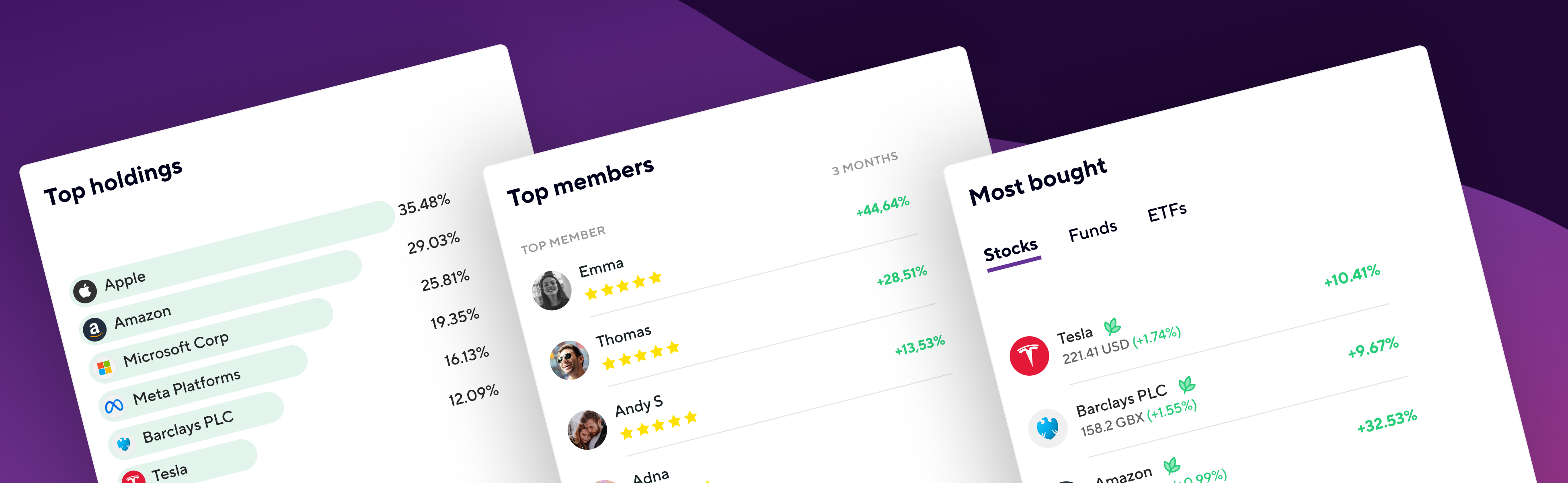
Elevate your platform's functionality with our robust React components. Seamlessly integrate real-time data such as top community holdings, trending posts, or top-rated members directly into your site's architecture. Give users dynamic, up-to-the-minute content, enhancing engagement and facilitating organic growth. Simplified installation. Extensive customization options
Installation
Before installing the package, you need to configure access to the registry.
- Make sure you have access to the GitHub repository.
- Create a personal access token in Github. The token needs read access to packages.
- Create a .npmrc-file in the project:
//npm.pkg.github.com/:_authToken=<TOKEN>
@stockrepublic:registry=https://npm.pkg.github.com
Then, install the package with your preferred package manager.
npm install @stockrepublic/republic-components
General props
General props that exist for all widget components.
const widgetOptions = {
title: "The title" // Optional custom title, default exists for all widget.
link: {
url: "", // URL for CTA button
text: "Explore community" // title for link
},
requestOptions: {
baseUrl: "", // base_url for API-requests
headers: {} // Optional additional headers that are passed through each API request
},
style: {} // Custom CSS applied to the widget.
};
Widget components
Top holdings
Display holdings by top members.
import {TopHoldings} from "@stockrepublic/republic-components";
const Component = () => {
const link = {
url: "https://google.com",
text: "Explore community"
};
const requestOptions = {
baseUrl: "https://api.stockrepublic.io"
};
return (
<TopHoldings
link={link}
requestOptions={requestOptions}
/>
);
};´
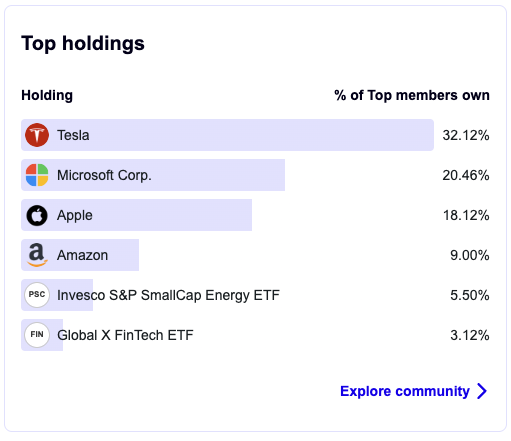
Most bought
Display most bought instruments.
import {MostBought} from "@stockrepublic/republic-components";
const Component = () => {
const link = {
url: "https://google.com",
text: "Explore community"
};
const requestOptions = {
baseUrl: "https://api.stockrepublic.io"
};
return (
<MostBought
link={link}
requestOptions={requestOptions}
/>
);
};
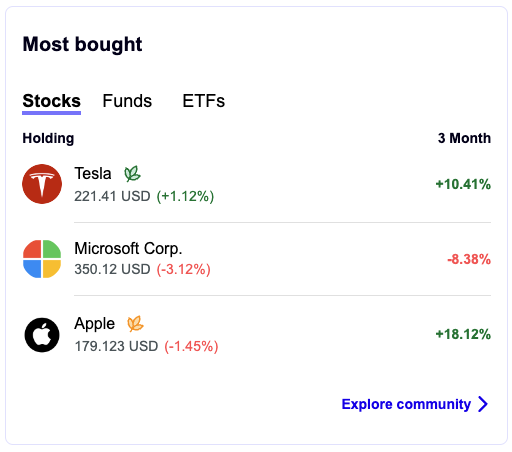
Top members
Display top members ranked by portfolio rating.
import {TopMembers} from "@stockrepublic/republic-components";
const Component = () => {
const link = {
url: "https://google.com",
text: "Go to web!"
};
const requestOptions = {
baseUrl: "https://api.stockrepublic.io"
};
return (
<TopMembers
link={link}
requestOptions={requestOptions}
/>
);
};
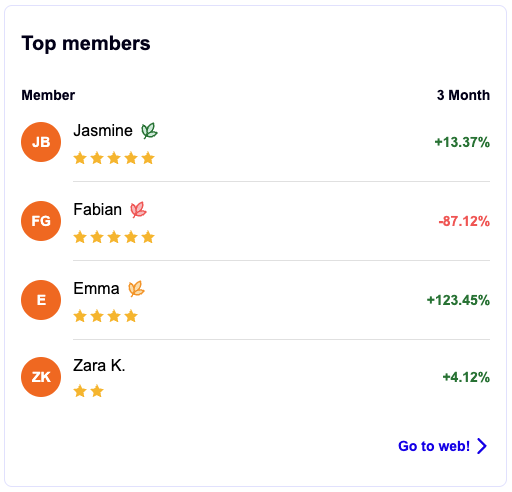
Popular posts
Display popular posts from the community.
import {PopularPosts} from "@stockrepublic/republic-components";
const Component = () => {
const link = {
url: "https://google.com",
text: "Explore community"
};
const requestOptions = {
baseUrl: "https://api.stockrepublic.io"
};
return (
<PopularPosts
link={link}
requestOptions={requestOptions}
/>
);
};
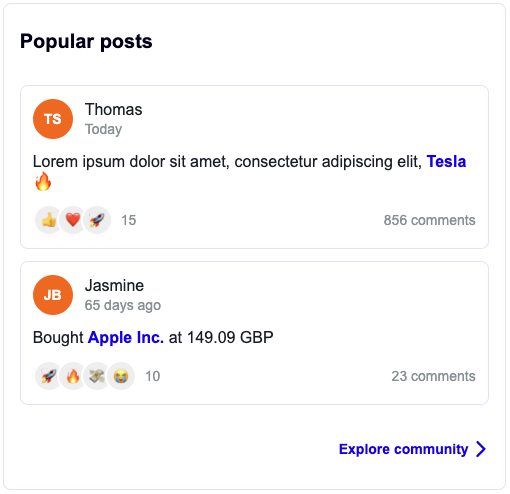
Customization Example
Example with custom font and title.
import {TopHoldings} from "@stockrepublic/republic-components";
const Component = () => {
const link = {
url: "https://google.com",
text: "The link text"
};
const requestOptions = {
baseUrl: "https://api.stockrepublic.io"
};
const style = {
fontFamily: "monospace"
};
const title = "Most popular holdings";
return (
<TopHoldings
title={title}
link={link}
requestOptions={requestOptions}
style={style}
/>
);
};
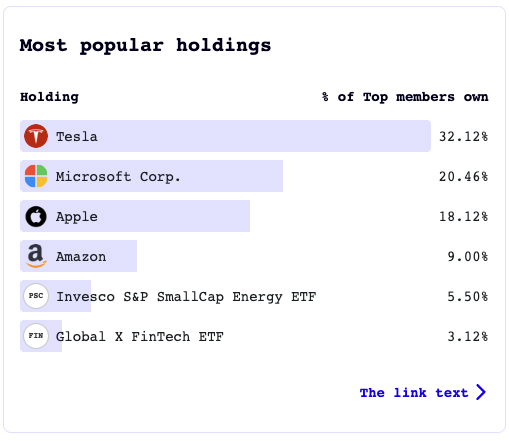
Updated 7 months ago